In this lesson, you learn more about how to show bitmaps.
In the computer world, a bitmap is simply a file containing an image. All your digital photos are bitmaps (although digital photos are typically much larger than the ones used in PsyToolkit). |
The standard "screen" size of PsyToolkit is 800 x 600 pixels. You can change this, but make sure that your bitmaps are always smaller than your screensize. |
Telling PsyToolkit which bitmap files to use
There is a "bitmaps" section. In this, you tell PsyToolkit which bitmaps to use. By default, PsyToolkit assumes that bitmaps are saved as PNG files. If you want a different format, you can tell PsyToolkit.
In the example below, I show you the bitmaps section with two files. The first one just has the name smiley, and PsyToolkit assumes there is a file smiley.png. In the case of house, you specify the jpeg filename.
bitmaps
smiley
house house.jpg
There are rules on the names: |
-
Filesnames are not allow to have spaces
-
Filenames cannot start with a number
-
Filenames cannot have special characters, such as the & sign.
Showing just a bitmap
The simplest command is just as this:
show bitmap smiley
Note, by default, the bitmap is shown at location 0,0, which is screen center.
Showing a bitmap at a specific location
You can add X/Y coordinates following the name of the bitmap.
show bitmap smiley 0 0
Of course, that is fairly pointless. But you can change 0 0 in anything.
Showing multiple bitmaps simultaneously
You can show bitmaps exactly at the same time by putting them between draw off and draw on.
draw off
show bitmap smiley -200 0
show bitmap house 200 0
draw on
Basically, as soon as PsyToolkit see draw off it will stop drawing the bitmaps until draw on is called. Without the commands draw off and draw on, the bitmpas would be shown one after another (there can be a 10 to 20 ms delay between them).
show bitmap smiley -200 0
show bitmap house 200 0
Showing a different bitmap in different conditions
Of course, you often want to show a different bitmap depending on the experimental condition you are in. In PsyToolkit, you can create a table with the different conditions. For example, you can show one of three different bitmaps in different trials.
A table with conditions
First, make sure you create a table with for each condition one line. In the example below, we have three conditions. We have three different bitmaps (smiley, house, and tree). We show them on different locations as well.
table mytable
smiley -200 0
house 200 0
tree 0 0
What you put in a table is entirely up to you. The example below has 3 columns, but you can have as many as you want, and you can decide whatever information you put in it (numbers, strings, stimuli names, etc). |
Refering to the table
By default, PsyToolkit choose a table row at random each time it carries out a task (you can change this default behaviour, as is explained elsewhere).
In the task, you know only one table row is being used. You refer to the columns with the @ sign. Thus @1 refers to the first column, @2 to the second, etc.
Thus if you want to show a bitmap at positions x y, you do it as follows:
show bitmap @1 @2 @3
This can mean that you show smiley at coordinates -200 0, or that you show the house at 200 0, etc.
Using bitmaps in animation
Sometimes, you want to capture people’s attention with animated stimuli. For example, if you want that people really look at the fixation point, you can animate it.
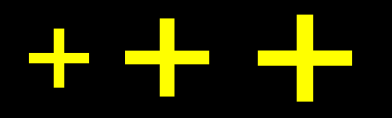
Make sure that you have bitmaps fixpoint1, fixpoint2, and fixpoint3. Fixpoint1 is smallest and Fixpoint3 is largest (see image above).
show bitmap fixpoint1
delay 80
show bitmap fixpoint2
delay 80
show bitmap fixpoint3
delay 80
clear 1 2 3
Load bitmaps from the internet
Instead of uploading bitmap files on PsyToolkit, you can also put them somewhere else. For this you need the complete URL of the file.
In the example below, we take the image tree from dropbox. In this case, note the ending on ?raw=1. This is important (you can also use dl=1 instead). |
bitmaps
tree https://www.dropbox.com/s/aunobj8stq3tb3u/tree.png?raw=1
Changing the coordinate system
By default X/Y coordinate refers to the center of the screen. You can change this using the option "origin". If you set it to "topleft", the coordinates 0 0 refer to the top left corner of the screen.
options
origin topleft
Running the code
This "task" is not really a task, because no keypresses are required. All we do here is just show various bitmaps. |
The downloading of the images can be a bit slow, because Dropbox is involved. Normally, starting up an experiment is much faster. But if you have very large images, Dropbox might be better. Try out what works best for you. |
The complete file
bitmaps
smiley
house house.jpg
tree https://www.dropbox.com/s/aunobj8stq3tb3u/tree.png?raw=1
fixpoint1
fixpoint2
fixpoint3
table mytable
smiley -200 0
house 200 0
tree 0 0
task mytask
table mytable
show bitmap fixpoint1
delay 80
show bitmap fixpoint2
delay 80
show bitmap fixpoint3
delay 80
clear 1 2 3
delay 100
show bitmap @1 @2 @3
delay 500
clear screen
block test
tasklist
mytask 10
end